Title: Basic PHP Tutorial for Beginners: A Step-by-Step Guide….
PHP, which stands for “Hypertext Preprocessor,” is a widely-used server-side scripting language designed for web development. It’s known for its simplicity, versatility, and the ability to integrate seamlessly with HTML. If you’re new to programming and want to start your journey with PHP, this easy-to-follow tutorial is the perfect place to begin. In this article, we will cover the basics of PHP, step by step, to help you get started.
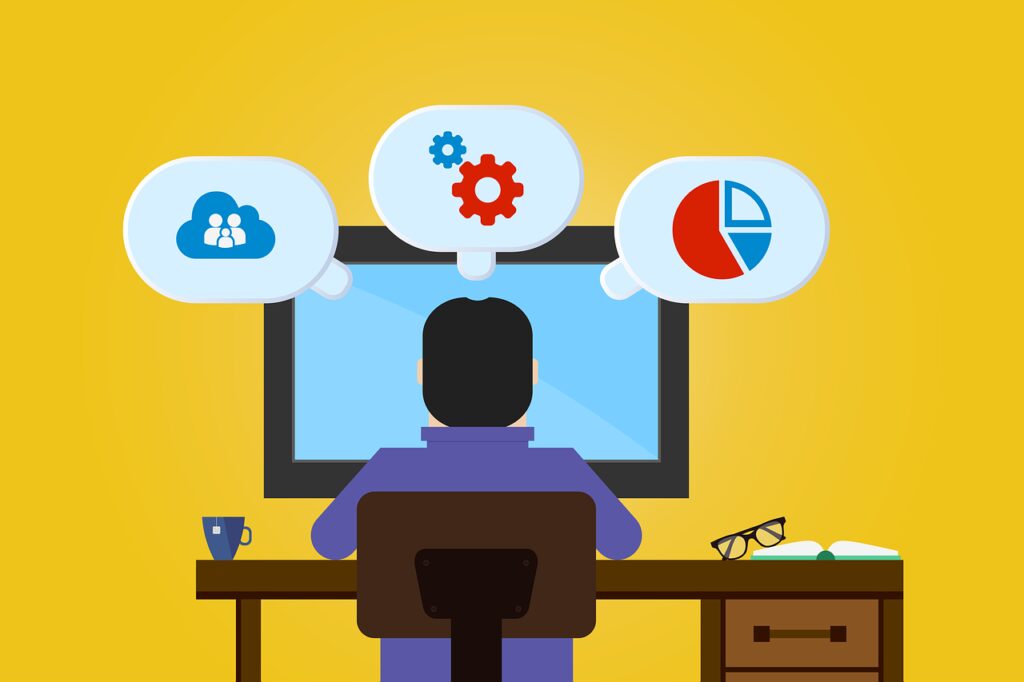
What is PHP?
PHP is a scripting language used for developing dynamic web pages and web applications. Unlike HTML, which is a markup language for creating static web content, PHP allows you to add functionality to your web pages, such as user authentication, form handling, and database interactions. PHP code is executed on the web server, and the results are sent to the web browser, making it an essential tool for web developers.
Setting Up Your Environment
Before diving into PHP, you’ll need a development environment to write and test your PHP scripts. Here’s a simple setup process:
- Choose a Code Editor: You can use any code editor or integrated development environment (IDE) to write PHP code. Some popular options include Visual Studio Code, PhpStorm, and Notepad++.
- Install a Local Server: To test your PHP scripts locally, you’ll need a local server environment. One of the most common options is XAMPP, which provides Apache (web server), MySQL (database), and PHP all in one package. Download and install XAMPP or a similar solution suitable for your operating system.
- Start the Server: After installing XAMPP, start the Apache server from the XAMPP Control Panel. This will allow you to run PHP scripts on your local machine.
- Create a Test Folder: Create a new folder in the ‘htdocs’ directory of your XAMPP installation. This folder will serve as the root directory for your PHP projects.
Writing Your First PHP Script
Let’s start by creating a simple PHP script to display a “Hello, World!” message. Follow these steps:
- Open your code editor and create a new file in your test folder. Save it with a .php extension, such as
hello.php
. - In the PHP file, you’ll start with the
<?php
tag to indicate the beginning of PHP code:
<?php
- Add the PHP code to display the message:
<?php
echo "Hello, World!";
?>
- Save the file.
Running Your PHP Script
Now that you’ve written your first PHP script, it’s time to see it in action:
- Open your web browser.
- In the address bar, type
http://localhost/your-folder-name/hello.php
, replacingyour-folder-name
with the name of the folder where you savedhello.php
. - Press Enter. You should see the “Hello, World!” message displayed in your browser.
Congratulations! You’ve successfully created and executed your first PHP script.
Basic PHP Syntax
Let’s explore some essential PHP syntax elements:
Variables
In PHP, you can declare variables using the $
symbol followed by the variable name. Variables in PHP are case-sensitive.
$name = "John";
$age = 30;
Comments
You can add comments to your PHP code to make it more understandable. PHP supports single-line and multi-line comments.
// This is a single-line comment
/*
This is a multi-line comment
It can span multiple lines
*/
Data Types
PHP supports various data types, including integers, floats, strings, booleans, arrays, and more. PHP dynamically assigns data types based on the value assigned to a variable.
$integer = 42;
$float = 3.14;
$string = "Hello, PHP!";
$boolean = true;
$array = [1, 2, 3];
Operators
PHP provides a wide range of operators for performing operations on variables and values, including arithmetic, comparison, and logical operators.
$a = 10;
$b = 5;
$sum = $a + $b; // Addition
$diff = $a - $b; // Subtraction
$product = $a * $b; // Multiplication
$quotient = $a / $b; // Division
$is_equal = ($a == $b); // Comparison (equal)
$is_greater = ($a > $b); // Comparison (greater than)
$and_result = ($a > $b) && ($a < 20); // Logical AND
$or_result = ($a > $b) || ($b < 3); // Logical OR
Conditional Statements and Loops
PHP allows you to control the flow of your program using conditional statements and loops.
If Statements
Use if
statements for decision-making in your code:
$age = 25;
if ($age < 18) {
echo "You are a minor.";
} elseif ($age >= 18 && $age < 65) {
echo "You are an adult.";
} else {
echo "You are a senior.";
}
Loops
PHP supports for
, while
, and foreach
loops for iterating through arrays and executing code repeatedly.
// For loop
for ($i = 1; $i <= 5; $i++) {
echo "Iteration $i<br>";
}
// While loop
$counter = 0;
while ($counter < 3) {
echo "Count: $counter<br>";
$counter++;
}
// Foreach loop (for arrays)
$colors = ["red", "green", "blue"];
foreach ($colors as $color) {
echo "Color: $color<br>";
}
Functions
Functions in PHP allow you to encapsulate blocks of code for reuse. Here’s how to define and use a function:
function greet($name) {
echo "Hello, $name!";
}
greet("Alice"); // Outputs "Hello, Alice!"
Conclusion
This basic PHP tutorial has introduced you to the fundamental concepts and syntax of PHP programming. From setting up your environment to writing your first PHP script and exploring essential PHP features, you’ve taken the first steps towards becoming a proficient PHP developer.
As you continue your PHP journey, consider exploring topics such as form handling, database connectivity, and more advanced PHP functions and libraries. There are numerous online resources, tutorials, and documentation available to help you deepen your PHP knowledge. Happy coding!
Pretty Gaming
สมัครเล่นเกมไพ่ออนไลน์ บาคาร่า sa casino
เว็บพนัน บาคาร่า คาสิโนออนไลน์ ไม่ผ่านเอเย่นต์
Hi, kam dashur të di çmimin tuaj
Can I contact Administration?
It is about advertisement on your website.
Thank.
Start your day with a pancake delivery from Mr. Pancake in Munich. Order now and enjoy a breakfast that’s not only convenient but also incredibly delicious. Pancakes
Can someone answer how to change the password ??
Maybe I’m doing something wrong?
Please help.
Yours faithfully.
Aloha, makemake wau eʻike i kāu kumukūʻai.
301 Moved Permanently More info…
View https://enshrouded-download.com/
Hey!
Who understands Bing algoritms these days? I am checking on serp for prompt same as 1 win apk variety platforms that I searched on search in top 1-10 are very good and developed and have very less links. What is their trick to rank that nice?
Hi, roeddwn i eisiau gwybod eich pris.
Здравейте, исках да знам цената ви.
Hi, მინდოდა ვიცოდე თქვენი ფასი.
Szia, meg akartam tudni az árát.
Hej, jeg ønskede at kende din pris.
হাই, আমি আপনার মূল্য জানতে চেয়েছিলাম.
Treat yourself to a pancake feast in Munich! Order delivery from Mr. Pancake and make your breakfast a memorable experience with a variety of delicious options. wolt Pancakes
Hola, quería saber tu precio..
Sveiki, es gribēju zināt savu cenu.