Title: The Basics of C Language: A Beginner’s Guide
The C programming language is often regarded as the foundation of modern programming. Its simplicity, power, and versatility have made it an essential language for both beginners and experienced programmers. In this article, we will delve into the basics of C language, providing you with a comprehensive understanding to kickstart your programming journey.
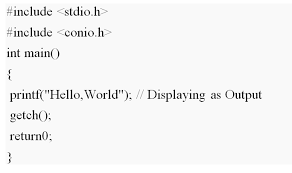
What is C Language?
C is a high-level, general-purpose programming language created by Dennis Ritchie in the early 1970s at Bell Labs. It has played a pivotal role in shaping the computing landscape and has inspired the development of many other programming languages. C is known for its efficiency, portability, and ability to handle low-level operations, making it ideal for system programming, embedded systems, and more.
Key Concepts in C Language
Before diving into the specifics, let’s explore some fundamental concepts in C:
1. Variables and Data Types
In C, you work with various data types like integers, floating-point numbers, characters, and more. You declare variables to store and manipulate data. For example:
int age = 25;
float price = 19.99;
char grade = 'A';
2. Operators
C provides a wide range of operators for performing operations on data. These include arithmetic operators (+, -, *, /), relational operators (==, !=, <, >), and logical operators (&&, ||, !). For example:
int x = 10;
int y = 5;
int sum = x + y; // sum contains 15
3. Control Structures
Control structures, like if statements, loops, and switch statements, are used to control the flow of a program. They help in making decisions and repeating actions. For example:
if (age >= 18) {
printf("You are an adult.\n");
} else {
printf("You are a minor.\n");
}
4. Functions
Functions are blocks of code that perform specific tasks. They allow you to modularize your code and reuse it efficiently. A basic function in C looks like this:
int add(int a, int b) {
return a + b;
}
5. Arrays and Pointers
Arrays are collections of similar data types, while pointers are variables that store memory addresses. Together, they enable efficient memory management and manipulation of data. For instance:
int numbers[5] = {1, 2, 3, 4, 5};
int *ptr = &numbers[0];
6. Input and Output
C provides functions like printf
and scanf
for input and output operations. These functions allow you to interact with the user and display information. For example:
printf("Enter your name: ");
char name[50];
scanf("%s", name);
printf("Hello, %s!\n", name);
The Structure of a C Program
A C program typically consists of functions. Every C program must have a main
function, which serves as the entry point. Here’s a basic structure:
#include <stdio.h>
int main() {
// Your code here
return 0;
}
The #include <stdio.h>
line is a preprocessor directive that includes the standard input/output library, allowing you to use functions like printf
and scanf
.
Compile and Run
To run a C program, you need to follow these steps:
- Write your C code in a text editor or an integrated development environment (IDE).
- Save the file with a
.c
extension (e.g.,myprogram.c
). - Open a terminal or command prompt and navigate to the folder containing your C file.
- Compile the code using a C compiler. For example, if you’re using the GCC compiler, you can compile your program with:
gcc myprogram.c -o myprogram
This command generates an executable file named myprogram
.
- Run the program:
./myprogram
Your program’s output will be displayed in the terminal.
Conclusion
This article has introduced you to the fundamental concepts of the C programming language. C’s simplicity, combined with its robust capabilities, makes it an excellent choice for anyone aspiring to become a programmer. As you delve deeper into C, you’ll discover its extensive libraries, advanced data structures, and its use in various domains, including system programming, game development, and more. Happy coding!
Hi, this is a comment.
To get started with moderating, editing, and deleting comments, please visit the Comments screen in the dashboard.
Commenter avatars come from Gravatar.
Please comment here